Design BookMyShow: An Online Movie Ticket Booking System
Get started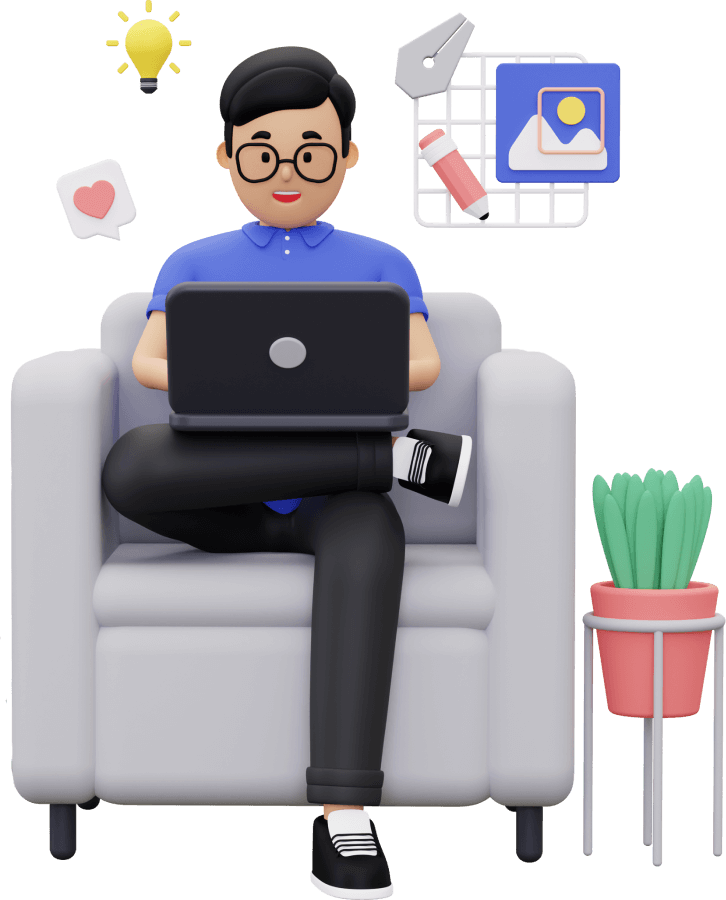
জয় শ্রী রাম
🕉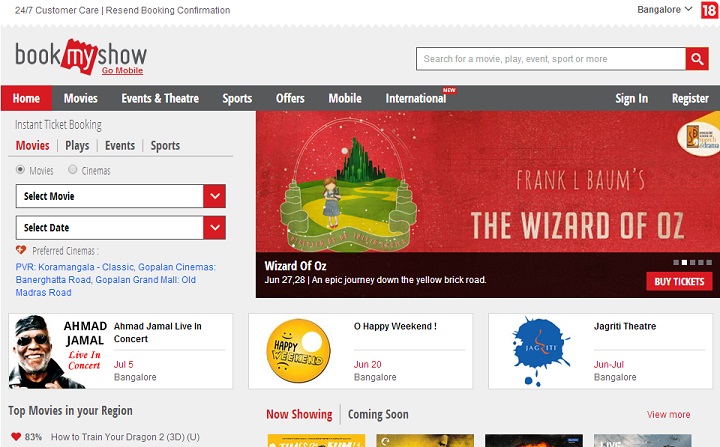
Problem Statement:
Design BookMyShow using Object Oriented principles.
Solution:
Object Oriented Analysis Framework:
A big part of the Object Oriented Design is Object Oriented Analysis. Object Oriented Analysis helps us figure out what are the classes we need to have and what all would be the responsibilities (i.e, methods and properties) of these classes. We would use the below step by step framework to efficiently handle any Object Oriented Design problem.-
Identifying the Actors. In most cases, each actor type would become a class.
-
Identifying the
uses cases of the system we are building and the user activities that
the actors will be engaged in.
-
Identifying all the Entities or Attributes that would eventually become the classes.
Before we can even use the framework, you need to ask clarifying questions to your interviewer to figure out what kind of system your interviewer wants you to design. Remember that your interviewer will intentionally keep the problem statement vague to see if you have the capability to gather the business requirements and analyse them to reach a viable design. In fact, what sets a great candidate apart from an average candidate is his/her ability to ask critical questions and analyse the requirements.
In this chapter, we will design a system like BookMyShow. The system would have a network of theater companies (like, big theater chains like AMC or Regal Cinemas or INOX , as well as small and regional movie theaters) in multiple cities and would contain all the information about movies currently playing and the ability to buy tickets for the available movie shows.
Now that we know what we are building, let's see how we can use our framework to come up with the Object Oriented Design for the system.
Step #1. Actors:
Let's think wh all are the ones that will using the system. The first class of users that come to mind are the movie watchers. Let's call them customrs. They will visit the website to buy movie tickets.Apart from buying tickets online, how else can they buy tickets ?
(1) Customers may call the customer care over the phone and make a booking.
(2) Customers may also just walk in and buy tickets from the cinema hall directly.
So, we have two more types of users who will be interacting with the system. They are:
-
Customer care representatives, whoc help customers make booking over the phone,
- Front Desk Assistants, who issue customers tickets at the cinema halls when a customer walks in and purchase a ticket. Front Desk Assistants are also responsible to check in the customers at the movie theater.
Also, someone from the company which owns the system needs to onboard the client theater companies in the system. We will call this person System Administrator.
It is important to understand how system administrator is different from theater administrator. Theater Administrators employees of the theater companies which are clients of the movie ticket booking system. So Theater Administrators will be employees of companies like AMC, INOX etc. Whereas System Administartors are employees of the company that owns the Online Movie Ticket Booking System, like BookMyShow, and are responsible for onboarding clients like AMC, INOX, Regal Cinemas etc on the system.
Step #2. User Activities and Use Cases of the System:
This is where you discuss the scope of your design with your interviewer. We would be focusing on the below use cases of the system:
-
System should be able to list the cities where affiliate cinemas are located.
-
Each theater company can have multiple movie halls and each movie hall can run multiple movie shows.
-
Once the customer selects a movie, the service should display the cinemas running that movie and its available shows.
-
The customer should be able to select a show at a particular theater and book their tickets.
-
The service should show the customer the different seat options available, like regular seats, premium seats,
ADA compliant accessible seats, recliner seats etc.
-
Customers should be able to pay with credit cards or cash.
- The system should ensure that no two customers can reserve the same seat.
Step #3. Entities:
- Theater Companies
- Theater Locations (Movie Hall / Cinema Hall / Theater / Multiplex)
- Movie
- Movie Show (a movie can can several shows in a cinema hall at different time or may be at the same time. Example: 2D and 3D version of a movie playing at the same time in two different shows at the same location.)
- Seat (a show will have seats for the movie watchers / customers.)
- Booking
- Payment
Object oriented Design and Class Implementation:
From our above analysis we get the below classes:
Actors: Customer, Customer Care Representative, Front Desk Assistant, Theater Administrator, System Administrator:
package OOD.TicketBookingSystem.User;
/**
* Created by Abhishek Dey on 10/29/21.
*/
public class Member {
private String id;
private String name;
private String email;
private String phone;
public Member(String id, String name, String email, String phone) {
this.id = id;
this.name = name;
this.email = email;
this.phone = phone;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getId() {
return id;
}
@Override
public boolean equals(Object o) {
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Member member = (Member) o;
return id.equals(member.id);
}
@Override
public int hashCode() {
return id.hashCode();
}
}
package OOD.TicketBookingSystem.User;
import OOD.TicketBookingSystem.Booking.Booking;
import java.util.ArrayList;
import java.util.List;
/**
* Created by Abhishek Dey on 10/29/21.
*/
public class Customer extends Member {
private List< Booking > currentBookings = new ArrayList<>();
public Customer(String name, String email, String phone) {
super(name, email, phone);
}
public boolean makeBooking(Booking booking) {
// making a booking for a movie show
// if booking goes through successfully:
// add the booking to currentBookings list
// currentBookings.add(booking);
// return true
// if the booking fails:
// return false;
}
public List< Booking > getBookings() {
return currentBookings;
}
public boolean cancelBooking(Booking booking) {
// if the cancelation go through
// remove the booking from currentBookings list and
// return true
//
// otherwise return false
}
}
package OOD.TicketBookingSystem.User;
import OOD.TicketBookingSystem.Booking.Booking;
/**
* Created by Abhishek Dey on 10/29/21.
*/
public class CustomerCareRepresentative extends Member {
public CustomerCareRepresentative(String id, String name, String email, String phone) {
super(id, name, email, phone);
}
public boolean createBooking(Booking booking) {
// book a movie as requested by a customer
}
}
package OOD.TicketBookingSystem.User;
import OOD.TicketBookingSystem.Booking.Booking;
/**
* Created by Abhishek on 10/29/21.
*/
public class FrontDeskAssistant extends Member {
public FrontDeskAssistant(String id, String name, String email, String phone) {
super(id, name, email, phone);
}
public boolean createBookingAndIssueTicket(Booking booking) {
// book a movie as requested by a customer
}
// check in a customer
public boolean checkIn(Booking booking) {
return booking.checkIn();
}
}
package OOD.TicketBookingSystem.User;
import OOD.TicketBookingSystem.Movie.Movie;
import OOD.TicketBookingSystem.Theater.Show;
import OOD.TicketBookingSystem.Theater.MovieHall;
import OOD.TicketBookingSystem.Theater.TheaterCompany;
/**
* Created by Abhishek Dey on 10/29/21.
*/
// Administrator of a Theater Chain or Cinema Hall Company or Multiplex Chain
// Example: Administrator of AMC / Regal Cinemas / INOX etc
public class TheaterAdministrator extends Member {
private TheaterCompany theaterCompany;
public TheaterAdministrator(String id, String name, String email, String phone) {
super(id, name, email, phone);
}
public boolean addMovie(Movie movie, MovieHall theater) {
theater.addMovie(movie);
}
public boolean addShow(Show show, MovieHall theater) {
theater.addShow(show);
}
}
package OOD.TicketBookingSystem.User;
import OOD.TicketBookingSystem.Theater.TheaterCompany;
import OOD.TicketBookingSystem.TicketBookingSystem;
/**
* Created by Abhishek Dey on 11/13/21.
*/
public class SystemAdministrator extends Member {
private TicketBookingSystem system;
public SystemAdministrator(TicketBookingSystem system) {
this.system = system;
}
public void addNewTheaterCompany(TheaterCompany theaterCompany) {
system.addTheaterCompany(theaterCompany);
}
public void removeTheaterCompany(TheaterCompany theaterCompany) {
system.removeClient(theaterCompany);
}
public TicketBookingSystem getSystem() {
return system;
}
public void setSystem(TicketBookingSystem system) {
this.system = system;
}
}
Theater Company:
package OOD.TicketBookingSystem.MovieHall;
import OOD.TicketBookingSystem.Movie.Movie;
import java.util.Set;
/**
* Created by Abhishek Dey on 10/29/21.
*/
public class TheaterCompany {
private String companyId;
private String name;
private java.util.Set< MovieHall > theatersOwned;
private Address headQuarter;
public TheaterCompany(String companyId, String name, Address headQuarter) {
this.companyId = companyId;
this.name = name;
this.headQuarter = headQuarter;
}
public void addTheater(MovieHall theater) {
theatersOwned.add(theater);
}
public void shutdownTheater(MovieHall theater) {
theatersOwned.remove(theater);
}
public String getCompanyId() {
return companyId;
}
public void updateCompanyId(String companyId) {
this.companyId = companyId;
}
public String getName() {
return name;
}
public void updateName(String name) {
this.name = name;
}
public Set< MovieHall > getTheatersOwned() {
return theatersOwned;
}
public Address getHeadQuarter() {
return headQuarter;
}
public void updateHeadQuarter(Address headQuarter) {
this.headQuarter = headQuarter;
}
}
Movie Hall:
package OOD.TicketBookingSystem.MovieHall;
import OOD.TicketBookingSystem.Movie.Movie;
import java.util.HashSet;
import java.util.Set;
/**
* Created by Abhishek Dey on 10/29/21.
*/
// This class encapsulates cinema hall or theater (depending on
// which country you live in you may have different terms for theater or cinema hall or multiplex)
public class MovieHall {
private TheaterCompany owningCompany;
private String name;
private Set< Show > shows = new HashSet<>();
private Set< Movie > moviesCurrentlyPlaying = new HashSet<>();
public MovieHall(TheaterCompany owningCompany, String name, Set shows, Set moviesCurrentlyPlaying) {
this.owningCompany = owningCompany;
this.name = name;
}
public void addShow(Show show) {
shows.add(show);
}
public void addMovie(Movie movie) {
moviesCurrentlyPlaying.add(movie);
}
public boolean removeMovie(Movie movie) {
if (!shows.contains(movie)) {
moviesCurrentlyPlaying.remove(movie);
return true;
}
return false;
}
public boolean removeShow(Show show) {
if (!shows.contains(show)) {
shows.remove(show);
return true;
}
return false;
}
}
Movie Show:
package OOD.TicketBookingSystem.Theater;
import OOD.TicketBookingSystem.Movie.Movie;
/**
* Created by Abhishek Dey on 10/29/21.
*/
// This class encapsulates a Movie Show
public class Show {
private int showId;
private long createdOn;
private String startTime; // example: 6:30PM
private int durationInMin;
private MovieHall playingAt;
private Movie movie;
public Show(int showId, String startTime, MovieHall playingAt, Movie movie) {
this.showId = showId;
this.createdOn = System.currentTimeMillis();
this.startTime = startTime;
this.playingAt = playingAt;
this.movie = movie;
this.durationInMin = movie.getDurationInMins();
}
public int getShowId() {
return showId;
}
public void setShowId(int showId) {
this.showId = showId;
}
public long getCreatedOn() {
return createdOn;
}
public void setCreatedOn(long createdOn) {
this.createdOn = createdOn;
}
public String getStartTime() {
return startTime;
}
public void setStartTime(String startTime) {
this.startTime = startTime;
}
public int getDurationInMin() {
return durationInMin;
}
public void setDurationInMin(int durationInMin) {
this.durationInMin = durationInMin;
}
public MovieHall getPlayingAt() {
return playingAt;
}
public void setPlayingAt(MovieHall playingAt) {
this.playingAt = playingAt;
}
public Movie getMovie() {
return movie;
}
public void setMovie(Movie movie) {
this.movie = movie;
}
}
Movie Theater Seat:
package OOD.TicketBookingSystem.Theater;
/**
* Created by Abhishek Dey on 10/29/21.
*/
public class Seat {
public enum SeatType {
REGULAR, PREMIUM, ACCESSIBLE, RECLINER
}
private int seatId;
private boolean isReserved;
private double price;
private Show show;
public boolean book() {
if (!isReserved) {
isReserved = true;
return true;
}
return false;
}
public void markAsAvailable() {
isReserved = false;
}
public int getSeatId() {
return seatId;
}
public boolean isReserved() {
return isReserved;
}
public double getPrice() {
return price;
}
public void updatePrice(double price) {
this.price = price;
}
public Show getShow() {
return show;
}
public void updateShow(Show show) {
this.show = show;
}
}
Location Information:
package OOD.TicketBookingSystem.MovieHall;
/**
* Created by Abhishek Dey on 10/29/21.
*/
public class Address {
private String streetAddress;
private String city;
private String state;
private String zipCode;
private String country;
public Address(String streetAddress, String city, String state, String zipCode, String country) {
this.streetAddress = streetAddress;
this.city = city;
this.state = state;
this.zipCode = zipCode;
this.country = country;
}
public String getStreetAddress() {
return streetAddress;
}
public void setStreetAddress(String streetAddress) {
this.streetAddress = streetAddress;
}
public String getCity() {
return city;
}
public void setCity(String city) {
this.city = city;
}
public String getState() {
return state;
}
public void setState(String state) {
this.state = state;
}
public String getZipCode() {
return zipCode;
}
public void setZipCode(String zipCode) {
this.zipCode = zipCode;
}
public String getCountry() {
return country;
}
public void setCountry(String country) {
this.country = country;
}
}
Movie:
package OOD.TicketBookingSystem.Movie;
import OOD.TicketBookingSystem.Theater.Show;
import OOD.TicketBookingSystem.User.TheaterAdministrator;
import java.util.ArrayList;
import java.util.List;
/**
* Created by Abhishek Dey on 10/29/21.
*/
public class Movie {
private String title;
private String description;
private int durationInMins;
private String language;
private long releaseDate;
private String genre;
private TheaterAdministrator movieAddedBy;
private List< Show > shows = new ArrayList<>();
public Movie(
String title,
String description,
int durationInMins,
String language,
long releaseDate,
String genre,
TheaterAdministrator movieAddedBy) {
this.title = title;
this.description = description;
this.durationInMins = durationInMins;
this.language = language;
this.releaseDate = releaseDate;
this.genre = genre;
this.movieAddedBy = movieAddedBy;
}
public String getTitle() {
return title;
}
public void updateTitle(String title) {
this.title = title;
}
public String getDescription() {
return description;
}
public void updateDescription(String description) {
this.description = description;
}
public int getDurationInMins() {
return durationInMins;
}
public void updateDurationInMins(int durationInMins) {
this.durationInMins = durationInMins;
}
public String getLanguage() {
return language;
}
public void updateLanguage(String language) {
this.language = language;
}
public long getReleaseDate() {
return releaseDate;
}
public void updateReleaseDate(long releaseDate) {
this.releaseDate = releaseDate;
}
public String getGenre() {
return genre;
}
public void updateGenre(String genre) {
this.genre = genre;
}
public TheaterAdministrator getMovieAddedBy() {
return movieAddedBy;
}
public void updateMovieAddedBy(TheaterAdministrator movieAddedBy) {
this.movieAddedBy = movieAddedBy;
}
public List< Show > getShows() {
return shows;
}
public void adShow(Show show) {
shows.add(show);
}
}
Booking:
package OOD.TicketBookingSystem.Booking;
import OOD.TicketBookingSystem.Theater.Show;
import OOD.TicketBookingSystem.Theater.Seat;
import java.util.List;
/**
* Created by Abhishek Dey on 10/29/21.
*/
public class Booking {
public enum BookingStatus {
PENDING, CONFIRMED, CHECKED_IN, CANCELED
}
private String bookingNumber;
private long createdOn;
private BookingStatus status;
private Show show;
private List< Seat > seats;
private Payment payment;
public Booking(String bookingNumbe, Show show, List< Seat > seats, Payment payment) {
this.bookingNumber = bookingNumber;
this.createdOn = System.currentTimeMillis();
this.status = BookingStatus.PENDING;
this.show = show;
this.seats = seats;
this.payment = payment;
}
public boolean makePayment(Payment payment) {
// if payments succeeds
// this.payment = payment;
// return true;
// if paymeng is unsuccessful:
// return false;
}
public boolean cancel() {
if (status != BookingStatus.CHECKED_IN) {
status = BookingStatus.CANCELED;
return true;
}
return false;
}
public boolean reserveSeat(Seat seat) {
return seat.book();
}
public boolean reserveSeats(List< Seat > requestedSeats) {
for (Seat seat : requestedSeats) {
if (!seat.book()) {
unreserve(seats); // if we are not able to
// reserve all the requested seats we do NOT do partial booking
return false;
}
}
return true;
}
private void unreserve(List< Seat > seats) {
for (Seat seat : seats) {
if (!seat.isReserved()) {
return;
}
seat.markAsAvailable();
}
}
public boolean confirmBooking() {
if (status == BookingStatus.PENDING) {
status = BookingStatus.CONFIRMED;
return true;
}
return false;
}
public boolean checkIn() {
if (status == BookingStatus.CONFIRMED) {
status = BookingStatus.CHECKED_IN;
return true;
}
return false;
}
}
Payment:
package OOD.TicketBookingSystem.Booking;
/**
* Created by Abhishek Dey on 10/29/21.
*/
public class Payment {
public enum PaymentStatus {
UNPAID, PENDING, COMPLETED, DECLINED, CANCELLED, REFUNDED
}
public enum PaymentMethod {
CASH, CREDIT_CARD
}
private double amount;
private long createdOn;
private int transactionId;
private PaymentStatus status;
private PaymentMethod paymentMethod;
public Payment(double amount, int transactionId, PaymentStatus status, PaymentMethod paymentMethod) {
this.amount = amount;
this.createdOn = System.currentTimeMillis();
this.transactionId = transactionId;
this.paymentMethod = paymentMethod;
}
public void updatePauymentStatus(PaymentStatus status) {
this.status = status;
}
public double getAmount() {
return amount;
}
public long getCreatedOn() {
return createdOn;
}
public int getTransactionId() {
return transactionId;
}
public PaymentStatus getStatus() {
return status;
}
public PaymentMethod getPaymentMethod() {
return paymentMethod;
}
public void setPaymentMethod(PaymentMethod paymentMethod) {
this.paymentMethod = paymentMethod;
}
}
Python code:
class Member:
def __init__(self, id, name, email, phone):
self._id = id
self._name = name
self._email = email
self._phone = phone
def getName(self):
return self._name
def setName(self, name):
self._name = name
def getEmail(self):
return self._email
def setEmail(self, email):
self._email = email
def getPhone(self):
return self._phone
def setPhone(self, phone):
self._phone = phone
def getId(self):
return self._id
def equals(self, o):
if self is o:
return True
if o is None or type(o) != Member:
return False
member = o
return self._id == member._id
def hashCode(self):
return hash(self._id)
--------------------------------------------------
from OOD.TicketBookingSystem.Member import Member
class Customer(Member):
def __init__(self, name, email, phone):
self._currentBookings = []
id = 0
super().__init__(id, name, email, phone)
def makeBooking(self, booking):
pass
def getBookings(self):
return self._currentBookings
def cancelBooking(self, booking):
pass
----------------------------------------------------------
from OOD.TicketBookingSystem.Member import Member
class FrontDeskAssistant(Member):
def __init__(self, id, name, email, phone):
super().__init__(id, name, email, phone)
def createBookingAndIssueTicket(self, booking):
pass
def checkIn(self, booking):
return booking.checkIn()
-----------------------------------------------------------
from OOD.TicketBookingSystem.Member import Member
class TheaterAdministrator(Member):
def __init__(self, id, name, email, phone):
self._theaterCompany = None
super().__init__(id, name, email, phone)
def addMovie(self, movie, theater):
theater.addMovie(movie)
def addShow(self, show, theater):
theater.addShow(show)
-----------------------------------------------------------
from OOD.TicketBookingSystem.Member import Member
class SystemAdministrator(Member):
def __init__(self, system, id, name, email, phone):
super().__init__(id, name, email, phone)
self._system = system
def addNewTheaterCompany(self, theaterCompany):
self._system.addTheaterCompany(theaterCompany)
def removeTheaterCompany(self, theaterCompany):
self._system.removeClient(theaterCompany)
def getSystem(self):
return self._system
def setSystem(self, system):
self._system = system
-----------------------------------------------------------
from OOD.TicketBookingSystem.Member import Member
class SystemAdministrator(Member):
def __init__(self, system, id, name, email, phone):
super().__init__(id, name, email, phone)
self._system = system
def addNewTheaterCompany(self, theaterCompany):
self._system.addTheaterCompany(theaterCompany)
def removeTheaterCompany(self, theaterCompany):
self._system.removeClient(theaterCompany)
def getSystem(self):
return self._system
def setSystem(self, system):
self._system = system
-----------------------------------------------------------
class MovieHall:
def __init__(self, owningCompany, name, shows, moviesCurrentlyPlaying):
self._owningCompany = None
self._name = None
self._shows = set()
self._moviesCurrentlyPlaying = set()
self._owningCompany = owningCompany
self._name = name
def addShow(self, show):
self._shows.add(show)
def addMovie(self, movie):
self._moviesCurrentlyPlaying.add(movie)
def removeMovie(self, movie):
if movie not in self._shows:
self._moviesCurrentlyPlaying.remove(movie)
return True
return False
def removeShow(self, show):
if show not in self._shows:
self._shows.remove(show)
return True
return False
-----------------------------------------------------------
import time
class Show:
def __init__(self, showId, startTime, playingAt, movie):
self._showId = showId
self._createdOn = round(time.time() * 1000)
self._startTime = startTime
self._playingAt = playingAt
self._movie = movie
self._durationInMin = movie.getDurationInMins()
def getShowId(self):
return self._showId
def setShowId(self, showId):
self._showId = showId
def getCreatedOn(self):
return self._createdOn
def setCreatedOn(self, createdOn):
self._createdOn = createdOn
def getStartTime(self):
return self._startTime
def setStartTime(self, startTime):
self._startTime = startTime
def getDurationInMin(self):
return self._durationInMin
def setDurationInMin(self, durationInMin):
self._durationInMin = durationInMin
def getPlayingAt(self):
return self._playingAt
def setPlayingAt(self, playingAt):
self._playingAt = playingAt
def getMovie(self):
return self._movie
def setMovie(self, movie):
self._movie = movie
----------------------------------------------------
from enum import Enum
class SeatType(Enum):
REGULAR = 0
PREMIUM = 1
ACCESSIBLE = 2
RECLINER = 3
class Seat:
def __init__(self):
self._seatId = 0
self._isReserved = False
self._price = 0
self._show = None
def book(self):
if not self._isReserved:
self._isReserved = True
return True
return False
def markAsAvailable(self):
self._isReserved = False
def getSeatId(self):
return self._seatId
def isReserved(self):
return self._isReserved
def getPrice(self):
return self._price
def updatePrice(self, price):
self._price = price
def getShow(self):
return self._show
def updateShow(self, show):
self._show = show
-----------------------------------------------------
class Address:
def __init__(self, streetAddress, city, state, zipCode, country):
self._streetAddress = streetAddress
self._city = city
self._state = state
self._zipCode = zipCode
self._country = country
def getStreetAddress(self):
return self._streetAddress
def setStreetAddress(self, streetAddress):
self._streetAddress = streetAddress
def getCity(self):
return self._city
def setCity(self, city):
self._city = city
def getState(self):
return self._state
def setState(self, state):
self._state = state
def getZipCode(self):
return self._zipCode
def setZipCode(self, zipCode):
self._zipCode = zipCode
def getCountry(self):
return self._country
def setCountry(self, country):
self._country = country
-----------------------------------------------------
class Movie:
def __init__(self, title, description, durationInMins, language, releaseDate, genre, movieAddedBy):
self._shows = []
self._title = title
self._description = description
self._durationInMins = durationInMins
self._language = language
self._releaseDate = releaseDate
self._genre = genre
self._movieAddedBy = movieAddedBy
def getTitle(self):
return self._title
def updateTitle(self, title):
self._title = title
def getDescription(self):
return self._description
def updateDescription(self, description):
self._description = description
def getDurationInMins(self):
return self._durationInMins
def updateDurationInMins(self, durationInMins):
self._durationInMins = durationInMins
def getLanguage(self):
return self._language
def updateLanguage(self, language):
self._language = language
def getReleaseDate(self):
return self._releaseDate
def updateReleaseDate(self, releaseDate):
self._releaseDate = releaseDate
def getGenre(self):
return self._genre
def updateGenre(self, genre):
self._genre = genre
def getMovieAddedBy(self):
return self._movieAddedBy
def updateMovieAddedBy(self, movieAddedBy):
self._movieAddedBy = movieAddedBy
def getShows(self):
return self._shows
def adShow(self, show):
self._shows.append(show)
-------------------------------------------------
import time
from enum import Enum
class BookingStatus(Enum):
PENDING = 0
CONFIRMED = 1
CHECKED_IN = 2
CANCELED = 3
class Booking:
def __init__(self, bookingNumber, show, seats, payment):
self._bookingNumber = bookingNumber
self._createdOn = round(time.time() * 1000)
self._status = BookingStatus.PENDING
self._show = show
self._seats = seats
self._payment = payment
def makePayment(self, payment):
pass
def cancel(self):
if self._status != BookingStatus.CHECKED_IN:
self._status = BookingStatus.CANCELED
return True
return False
def reserveSeat(self, seat):
return seat.book()
def reserveSeats(self, requestedSeats):
for seat in requestedSeats:
if not seat.book():
self._unreserve(self._seats)
return False
return True
def _unreserve(self, seats):
for seat in seats:
if not seat.isReserved():
return
seat.markAsAvailable()
def confirmBooking(self):
if self._status == BookingStatus.PENDING:
self._status = BookingStatus.CONFIRMED
return True
return False
def checkIn(self):
if self._status == BookingStatus.CONFIRMED:
self._status = BookingStatus.CHECKED_IN
return True
return False
-----------------------------------------------------------------
import time
from enum import Enum
class PaymentStatus(Enum):
UNPAID = 0
PENDING = 1
COMPLETED = 2
DECLINED = 3
CANCELLED = 4
REFUNDED = 5
class PaymentMethod(Enum):
CASH = 0
CREDIT_CARD = 1
class Payment:
def __init__(self, amount, transactionId, status, paymentMethod):
self._status = status
self._amount = amount
self._createdOn = round(time.time() * 1000)
self._transactionId = transactionId
self._paymentMethod = paymentMethod
def updatePauymentStatus(self, status):
self._status = status
def getAmount(self):
return self._amount
def getCreatedOn(self):
return self._createdOn
def getTransactionId(self):
return self._transactionId
def getStatus(self):
return self._status
def getPaymentMethod(self):
return self._paymentMethod
def setPaymentMethod(self, paymentMethod):
self._paymentMethod = paymentMethod